Creating Layouts. Grid Basics
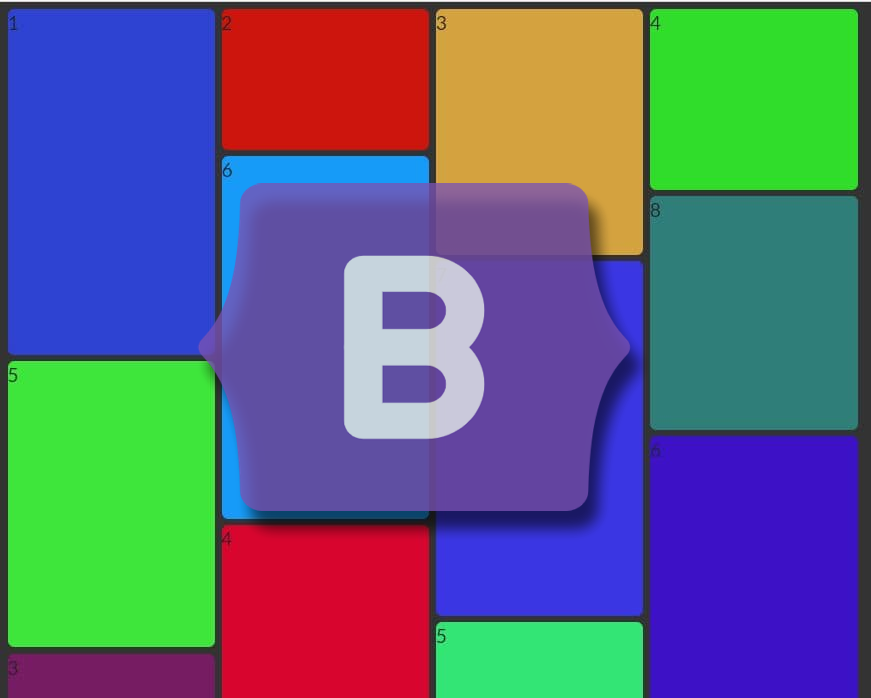
Throughout this mini-series, we will be learning how to create a great-looking website with Bootstrap 5.
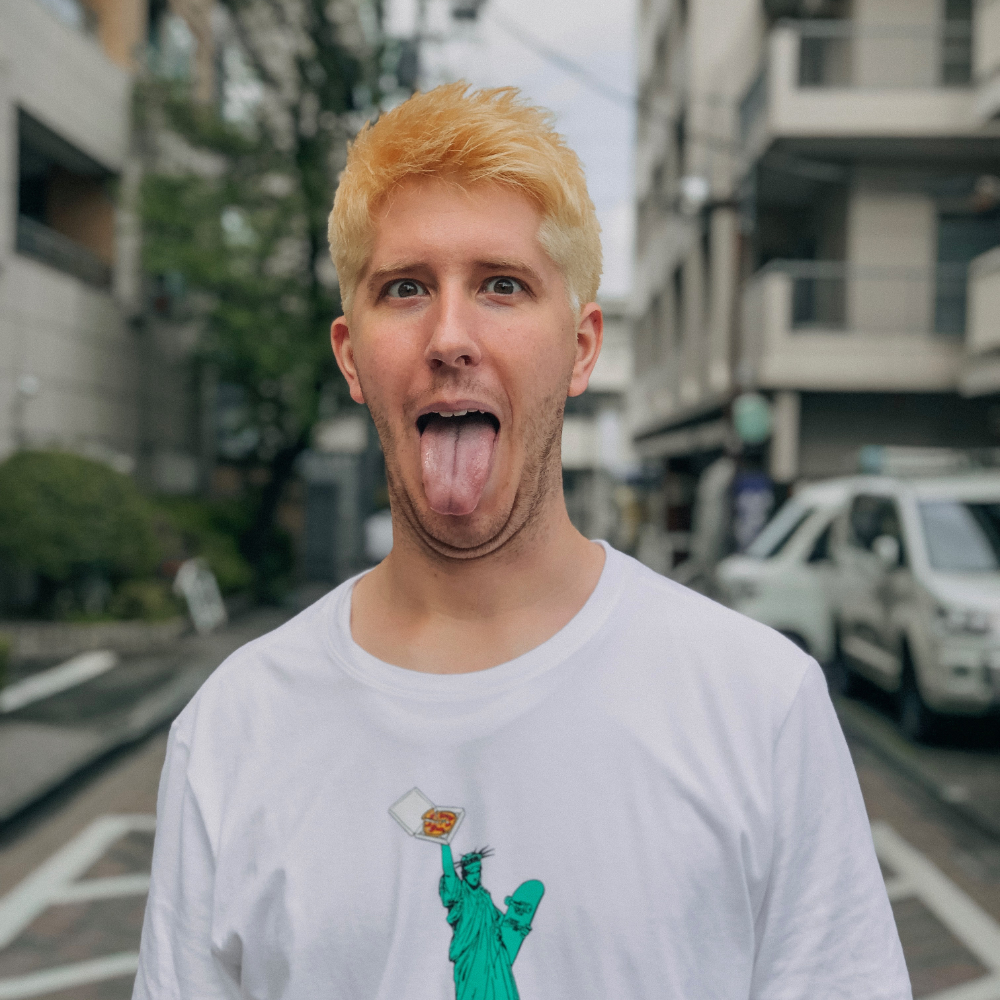
nicksensei
3 min read
Dec 19, 2020
Well, everyone, Bootstrap 5 has been officially launched. For those of you who may be unfamiliar, Bootstrap is the most popular framework for creating responsive, mobile-friendly websites.
Throughout this mini-series, we will be learning how to create a great-looking website with Bootstrap 5. To start off, we will be jumping into Bootstrap's grid system and learning how to layout a site. If this sounds like a cool project to you, then let's jump in and get started!
Getting Started
To get started with Bootstrap, we need to include Bootstrap in our HTML. Let's create a file called index.html
in our code editor where we will add Bootstrap. After creating index.html
, open the file and add Bootstrap by linking Bootstrap's CSS in our page's head
element and adding the JavaScript at the end of the body
tag, like this:
<! DOCTYPE html >
<html lang = "en">
<head>
<meta charset = "UTF-8">
<meta name = "viewport" content = "width = device-width, initial-scale = 1.0">
< title> Bootstrap 5 Mini-Series </title>
<! - Bootstrap CSS ->
<link href = "https://cdn.jsdelivr.net/npm/bootstrap@5.0.0-beta1/dist/css/bootstrap .min.css " rel =" stylesheet " integrity =" sha384-giJF6kkoqNQ00vy + HMDP7azOuL0xtbfIcaT9wjKHr8RbDVddVHyTfAAsrekwKmP1 " crossorigin ="anonymous ">
</head>
<body>
<h1> Bootstrap 5 Mini-Series </h1>
<! - Bootstrap JS ->
<script src = "https://cdn.jsdelivr.net/npm/bootstrap@5.0.0-beta1/dist/js/ bootstrap.bundle.min.js " integrity =" sha384-ygbV9kiqUc6oa4msXn9868pTtWMgiQaeYH7 / t7LECLbyPA2x65Kgf80OJFdroafW " crossorigin =" anonymous "> </script>
</body>
</html>
Perfect, Bootstrap is now incorporated into our project. Let's start laying out a webpage for a small carpentry business to learn how Bootstrap's grid system works.
Grid Basics
Bootstrap's grid system is mobile-first and built upon CSS FlexBox, allowing for all types of responsive layouts. A responsive design is the way elements align within a page at different screen sizes. The grid system consists of 3 key elements:
- Containers - an element with a class of
.container
used to contain and control a layout's width. - Rows - an element with a class of
.row
whose only function is a wrapper for columns. - Columns - an element with a class of
.col
used to divide the screen horizontally into columns.
Bootstrap's grid system follows this hierarchy: container --> row --> column. The container forms the base of the hierarchy, with each element nested inside the other.
That's enough theory—let's see it in action!
Services Section
For the services section, let's create a 3-column layout—one column for each service.
<section class="container">
<div class="row">
<div class="col-sm-12 col-md-4">
<h2>Framing</h2>
<p>Lorem ipsum dolor sit amet consectetur, adipisicing elit. Modi minus perspiciatis quis magni molestias id numquam, ea ab omnis iste.</p>
</div>
<div class="col-sm-12 col-md-4">
<h2>Decks</h2>
<p>Lorem, ipsum dolor sit amet consectetur adipisicing elit. Fuga ratione culpa vel autem porro dolor deleniti quibusdam eveniet, similique iste.</p>
</div>
<div class="col-sm-12 col-md-4">
<h2>Cabnets</h2>
<p>Lorem ipsum dolor, sit amet consectetur adipisicing elit. Dolorem, consequuntur numquam aliquam cumque voluptatibus nobis quas repudiandae quia excepturi iure.</p>
</div>
</div>
</section>
First, we start with the container by giving an element a class of .container
. Remember, containers are used to contain content and are the base of the grid system. Then inside the container, we will add a row with three columns. Lastly, we place our content inside each column.
Perfect, here's what mine looks like so far.