Running python 2 and python 3 on separate environments for the same Django project on Windows
Using venv and virtualenv in the same project
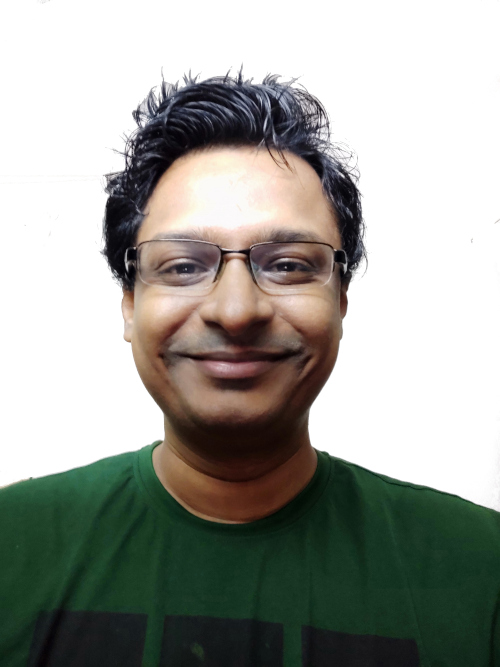
anjanesh
2 min read
Jul 13, 2022
Let's say your Django project name is ToDoWoo
Once you setup your Django project, there will be two `ToDoWoo` folders - one inside the other (which is the parent folder).
For Python 3 - download and install Python 3 from https://www.python.org/downloads/windows/ - as of today, the latest version is 3.10.5.
(What I am having on my Windows 10 laptop is Python 3.8.7 so I'll be referencing the path to 3.8)
"Python 3 itself comes with venv for managing environments which we will use for this guide." - https://docs.djangoproject.com/en/4.0/howto/windows/
So no need to do `pip install venv`
By default python's path should be at C:\Users\username\AppData\Local\Programs\Python\Python38
cd ToDoWoo
python -m venv project-env
.\ToDoWoo\Scripts\activate.bat
python .\manage.py runserver
For Python 2 - download and install Python 2.7.16 from https://www.python.org/downloads/release/python-2716/
We need to install a virtual envornment module that support Python 2 - since `venv` is a Python 3 specific module we have to install `virtualenv`
Assuming the default location of C:\Python27 :
cd ToDoWoo
C:\Python27\python.exe -m pip install virtualenv
C:\Python27\python.exe -m virtualenv py2
.\ToDoWoo\py2\Scripts\activate
python -m pip install Django==1.11.29
python .\manage.py runserver
Supporting both Python 2 and 3 in the same codebase was a common thing for several years.
https://discuss.python.org/t/using-code-for-python-versions-2-and-3-together-by-checking-version-number/17323
try:
from urllib.request import urlopen
except ImportError:
from urllib2 import urlopen
response = urlopen('https://jsonplaceholder.typicode.com/users')
json = response.read()
# print(json) # do something with json
response.close()