Using TailWind CSS 3.x with Django
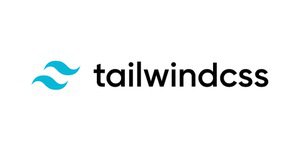
Getting started with TailWindCSS 3.0 in a Django Project without the use of nodeJS
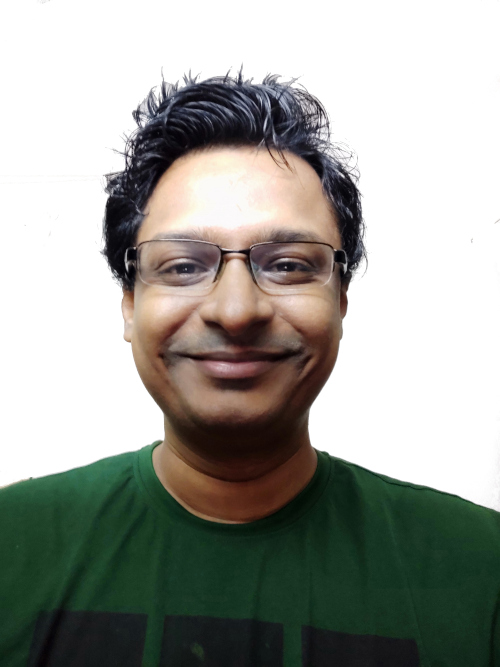
anjanesh
3 min read
Mar 12, 2022
I am going to assume you are aware of the regular django-admin startproject <project-name>
to set up a bare-bones Django app. Let’s assume the <project-name> is django-project and in python3 manage.py startapp <app-name>
app-name is todowoo.
I am also going to assume you know the basics of TailWindCSS as this tutorial is not about TailWind CSS but on the setup / tooling with Django without node.
TailWindCSS is a super new shiny CSS framework that has a whole bunch of utility classes that can be used to HTML tags to create beautiful looking web pages. But the number of utility classes is just too many. So many, that the version 2 of TailWind CSS file containing all the classes with their respective values is 2.8 MB - https://cdnjs.cloudflare.com/ajax/libs/tailwindcss/2.2.19/tailwind.min.css.
But the right way to include TailWindCSS is to run the TailWindCSS command to include only the classes which are used in your project and not add unused classes. This involves using npm : npm install -D tailwindcss - which is a JavaScript nodeJS module that needs to be run. There is a usual problem with this method - node_modules hell.
Since version 3.0, TailWind’s author has created a single executable of the nodeJS setup that can be run in the terminal directly by just downloading the executable - https://tailwindcss.com/blog/standalone-cli
Download the executable in your home directory from https://github.com/tailwindlabs/tailwindcss/releases/tag/v3.0.23 :
Example for macOS x64
$ curl -sLO https://github.com/tailwindlabs/tailwindcss/releases/download/v3.0.23/tailwindcss-macos-x64
$ chmod +x tailwindcss-macos-x64
$ mv tailwindcss-macos-x64 tailwindcss
Navigate to your app-name’s static folder, which in this case is
cd django-project/django-project/todowoo/static
$ touch tailwind.config.js
Edit tailwind.config.js using an editor like Visual Studio Code :
module.exports = {
content: ["../templates/**/*.{html,js}"],
theme: {
extend: {
colors: { gray: colors.slate, sky: colors.sky, },
},
},
plugins: [
require('@tailwindcss/forms')
],
}
This line is the most important when it comes to Django templates :
content: ["../templates/**/*.{html}"],
What this does, is that it scans all .html files within the templates directory and all sub-directories of templates for HTML classes and retains only those in the resultant styles.css file.
$touch style.src.css
Edit style.src.css using an editor like Visual Studio Code :
@tailwind base;
@tailwind components;
@tailwind utilities;
body
{
background-color:azure;
}
Now run this the terminal - you still have to be in django-project/todowoo/static
~/tailwindcss -i styles.src.css -o styles.css --minify
You can save the above line as styles.sh and run it in the terminal as `bash styles.sh` each time you edit the HTML template file(s) to include tailwind’s CSS in your HTML tags.
And then do the usual linking your styles.css in your todowoo/templates/base.html file or wherever you want to include it in.
<link rel="stylesheet" type="text/css" href="{% static 'styles.css' %}" />