Integrating Google Auth with Django Projects
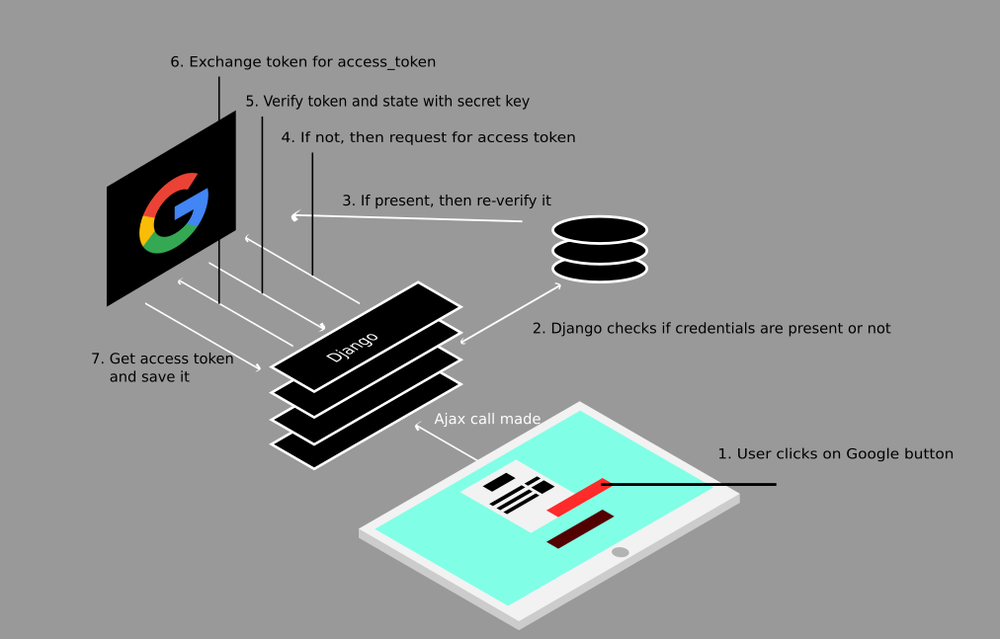
Make the authentication much easier for users.....
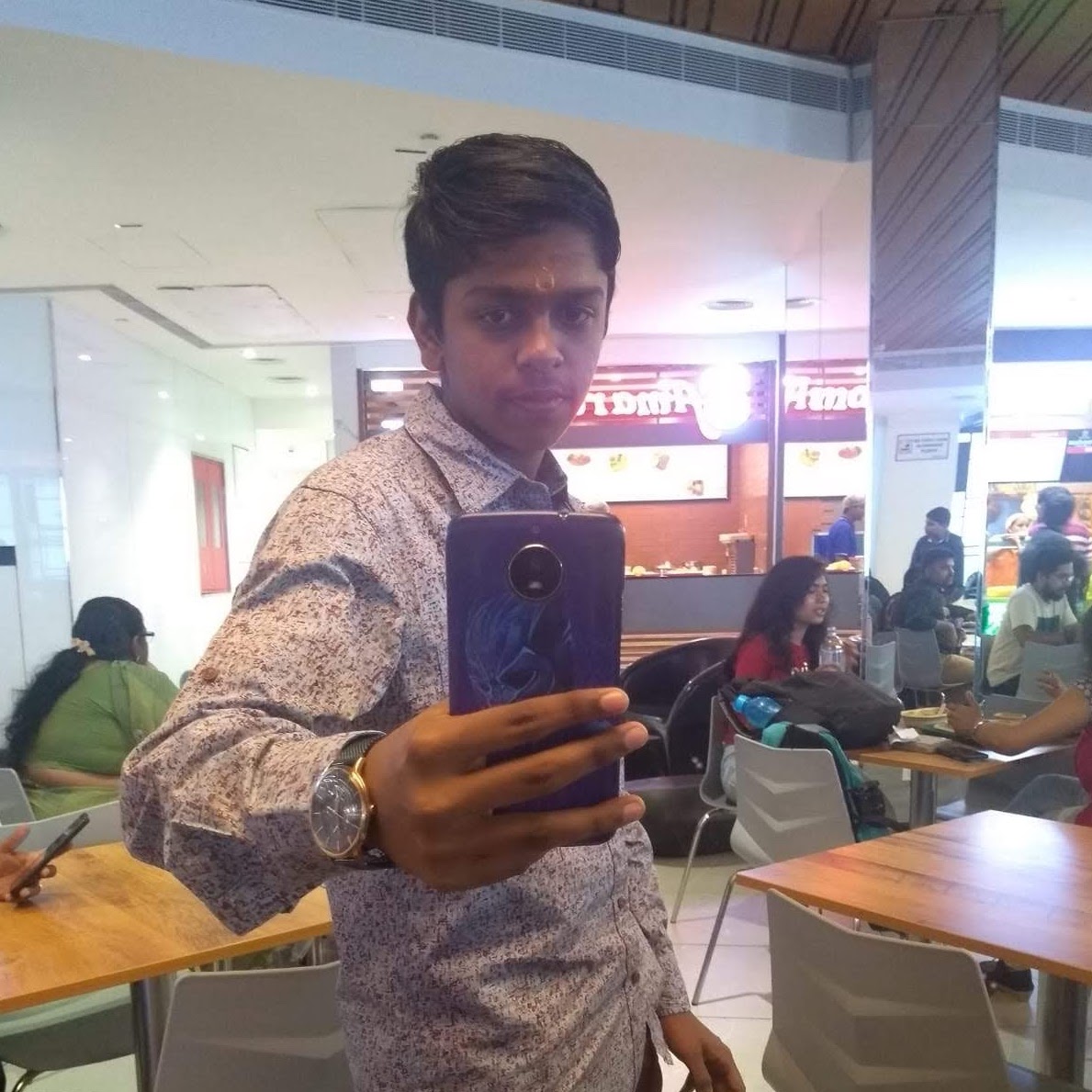
clashwithchiefrpjyt
3 min read
Oct 1, 2021
This blog is going to be about how to integrate social authentication with django projects.
This can be done in two ways either by using django-allauth or by using social-auth-app-django.
Let’s explore both the cases.
- Using django-allauth :
This serves a pretty powerful authentication service along with many providers for social authentication.
1. pip install django-allauth
2. In your settings.py :
AUTHENTICATION_BACKENDS = [
'django.contrib.auth.backends.ModelBackend',
'allauth.account.auth_backends.AuthenticationBackend',
]
INSTALLED_APPS = [
# The following apps are required:
'django.contrib.auth',
'django.contrib.messages',
'django.contrib.sites',
'allauth',
'allauth.account',
'allauth.socialaccount',
'allauth.socialaccount.providers.google',
#include all the providers you want to and refer all auth docs
]
SITE_ID = 1
SOCIALACCOUNT_PROVIDERS = {
'google': {
'APP': {
'client_id': your client id,
'secret': your secret,
'key': ''
}
}
}
How to get client_id and secret for the configurations ?
So go to https://console.cloud.google.com/ and create a new project and setup the oauth consent screen. After this go to credentials and create a new oauth client id.
After successful completion client_id and secret will be generated and add this to the required fields in settings.py.
3. In urls.py :
urlpatterns = [
...
path('accounts/', include('allauth.urls')),
…
]
iv In templates :
{% load socialaccount %}
<a href = “{% provider_login_url ‘google’ method = ‘oauth2’ %}”>Google</a>
v Cloud Platform Setup :
Inside the credentials setup the authorised javascript origins to http://127.0.0.1:8000 as well as http://localhost:8000.As for production mode type in the domain name.
Add Authorised redirect uri’s as the same given below
http://127.0.0.1:8000/accounts/google/login/callback/
The Cloud Platform looks like the image below
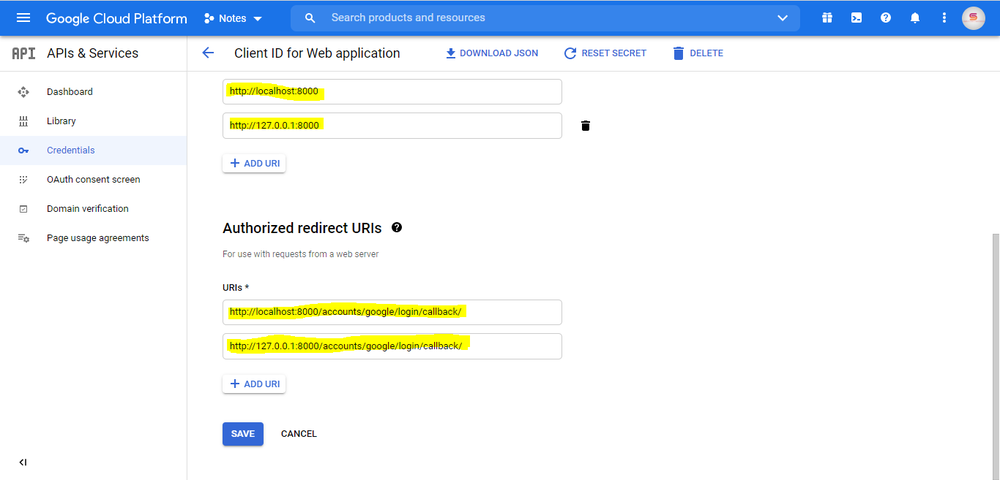
2) Using social-auth-app-django :
- Installation :
i) pip install social-auth-app-django
- Setup :
1. In settings.py :
INSTALLED_APPS = [
# The following apps are required:
'django.contrib.auth',
'django.contrib.messages',
'django.contrib.sites',
'social_django’,
#include all the providers you want to and refer all auth docs
]
SITE_ID = 1
AUTHENTICATION_BACKENDS = [
'django.contrib.auth.backends.ModelBackend',
'social_core.backends.google.GoogleOAuth2',
]
SOCIAL_AUTH_GOOGLE_OAUTH2_KEY = Your Client Id
SOCIAL_AUTH_GOOGLE_OAUTH2_SECRET = Your Client Secret
How to get client_id and secret for the configurations ?
So go to https://console.cloud.google.com/ and create a new project and setup the oauth consent screen. After this go to credentials and create a new oauth client id.
After successful completion client_id and secret will be generated and add this to the required fields in settings.py.
2. In urls.py :
urlpatterns = [
...
path('registration/', include('social_django.urls',namespace = “social”)),
…
]
3. In templates :
<a href = “{% url ‘social:begin’ ‘google-oauth2’ %}”>Google</a>
Inside the credentials setup the authorised javascript origins to http://127.0.0.1:8000 as well as http://localhost:8000.As for production mode type in the domain name.
Add Authorised redirect uri’s as the same given below
http://127.0.0.1:8000/registration/google/login/callback/
http://127.0.0.1:8000/registration/complete/google-oauth2/
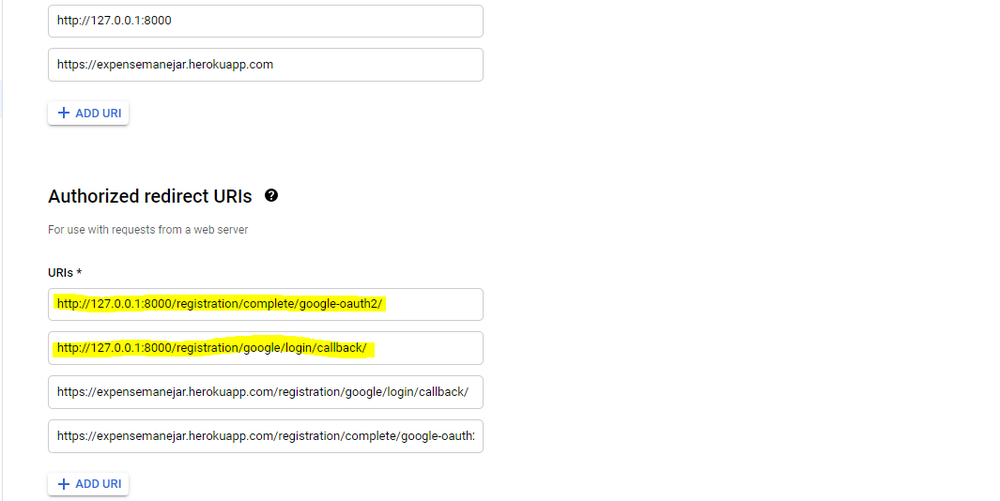
Guess this should set google authentication to work precisely. Have a great day !!!!.