Common SwiftUI List Error - Why Items Need to Be Identifiable and How to Fix It
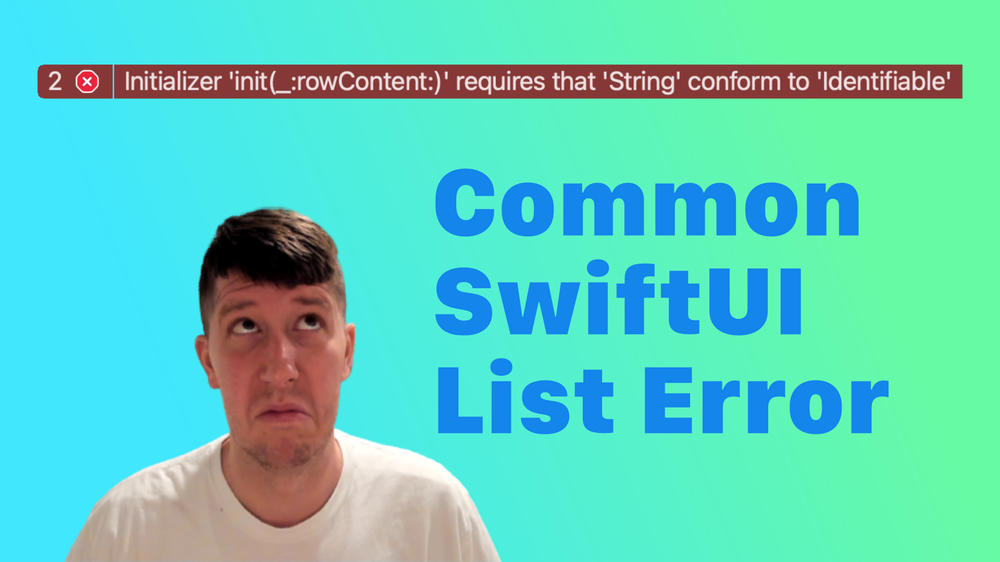
Ever get the error saying "Initializer requires that 'String' conform to 'Identifiable'"? This is why that is and how to fix it!
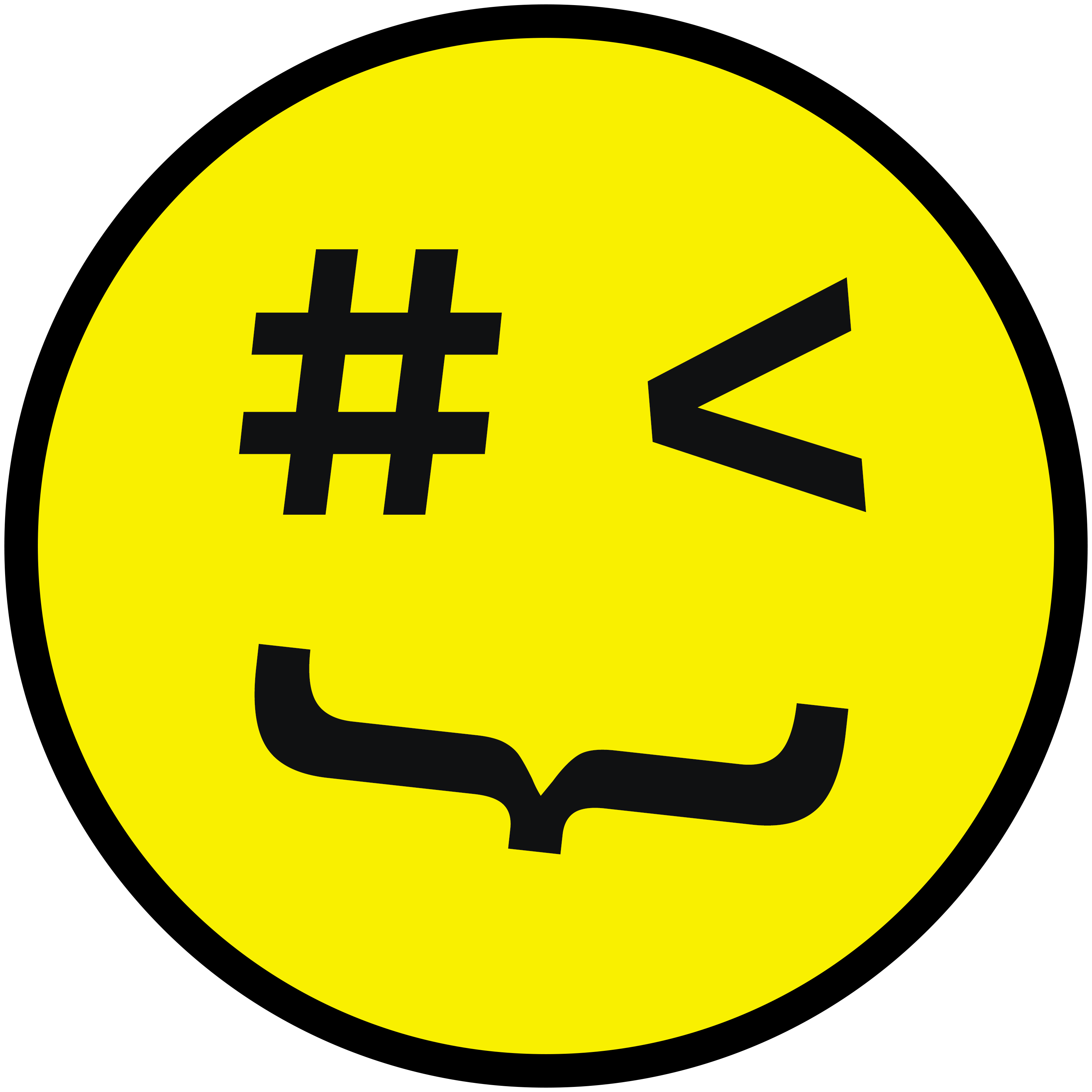
zappycode
1 min read
Oct 30, 2021
If you've ever wanted to list some strings or ints or something simple in SwiftUI, you know this error too well :)
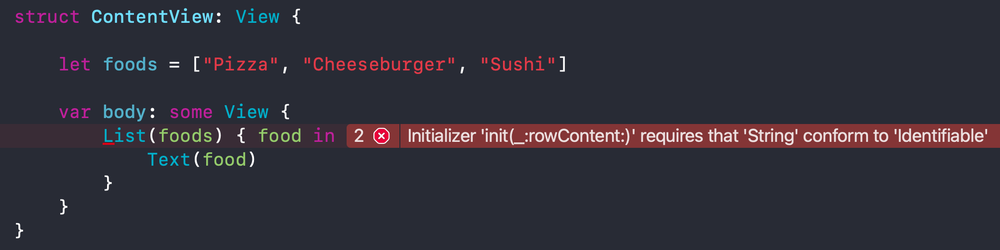
Well here's a video explaining why that error is showing up and how to fix it. I've even included a bonus tip on how to make objects for a list easier on yourself!
I learned about Lists from Paul's website and custom initializers from Dave and the CocoaHeads.
import SwiftUI
struct ContentView: View {
let foods = [Food(name: "Pizza"), Food(name: "Cheeseburger"), Food(name: "Sushi")]
var body: some View {
List(foods) { food in
Text(food.name)
}
}
}
struct Food: Identifiable {
var id: UUID
var name: String
init(name: String) {
id = UUID()
self.name = name
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}